1. Introduction
In this tutorial, you will learn how to use FastAPI to validate your data and generate interactive documentation for your web API using OpenAPI schema, Swagger UI, and ReDoc.
FastAPI is a modern, high-performance, web framework for building APIs with Python. It is based on standard Python type hints and the powerful Pydantic library. FastAPI provides several features that make it easy to create and document your web API, such as:
- Data validation: FastAPI validates the data you receive from clients and sends back meaningful error messages if the data is invalid.
- OpenAPI schema: FastAPI automatically generates an OpenAPI schema for your API, which describes the endpoints, parameters, responses, and models of your API.
- Swagger UI: FastAPI integrates with Swagger UI, a web-based tool that allows you to interact with your API and test its functionality.
- ReDoc: FastAPI also integrates with ReDoc, another web-based tool that provides a more elegant and customizable documentation for your API.
By the end of this tutorial, you will have a fully functional web API that validates your data and provides interactive documentation for your users. You will also learn how to customize the appearance and behavior of the documentation tools.
To follow this tutorial, you will need:
- Python 3.6 or higher installed on your machine.
- A code editor of your choice.
- A basic understanding of Python and web development.
Ready to get started? Let’s go!
2. What is FastAPI?
FastAPI is a modern, high-performance, web framework for building APIs with Python. It is based on standard Python type hints and the powerful Pydantic library. FastAPI provides several features that make it easy to create and document your web API, such as:
- Data validation: FastAPI validates the data you receive from clients and sends back meaningful error messages if the data is invalid.
- OpenAPI schema: FastAPI automatically generates an OpenAPI schema for your API, which describes the endpoints, parameters, responses, and models of your API.
- Swagger UI: FastAPI integrates with Swagger UI, a web-based tool that allows you to interact with your API and test its functionality.
- ReDoc: FastAPI also integrates with ReDoc, another web-based tool that provides a more elegant and customizable documentation for your API.
In this section, you will learn more about FastAPI and why it is a great choice for building web APIs. You will also learn how to install FastAPI and create a simple API with it.
Why FastAPI?
FastAPI is one of the fastest and most popular web frameworks for Python. It has many advantages over other frameworks, such as:
- Speed: FastAPI is built on top of Starlette, a lightweight ASGI framework that supports async and await syntax. This means that FastAPI can handle concurrent requests efficiently and scale well with high traffic.
- Simplicity: FastAPI is designed to be easy to use and learn. It follows the principle of “convention over configuration”, which means that you don’t have to write a lot of boilerplate code or configure complex settings. You just write your logic and FastAPI takes care of the rest.
- Documentation: FastAPI generates interactive documentation for your API automatically, based on the OpenAPI standard. You can use the documentation tools to explore and test your API without writing any extra code.
- Validation: FastAPI uses Pydantic to validate the data you receive from clients and send back to them. Pydantic is a library that allows you to define data models with type annotations and perform data validation and serialization. FastAPI integrates Pydantic seamlessly and gives you access to all its features.
These are just some of the reasons why FastAPI is a great framework for building web APIs. If you want to learn more about FastAPI and its features, you can visit the official website or the GitHub repository.
How to Install FastAPI?
To install FastAPI, you need to have Python 3.6 or higher installed on your machine. You can check your Python version by running the following command in your terminal:
python --version
If you don’t have Python installed, you can download it from the official website.
Once you have Python installed, you can install FastAPI and its dependencies using pip, a package manager for Python. To do so, run the following command in your terminal:
pip install fastapi[all]
This will install FastAPI along with all the optional dependencies, such as Starlette, Pydantic, Uvicorn, and the documentation tools. You can also install only the required dependencies by omitting the [all] part.
After installing FastAPI, you are ready to create your first API with it.
How to Create a Simple API with FastAPI?
To create a simple API with FastAPI, you need to follow these steps:
- Create a file named main.py and open it with your code editor.
- Import FastAPI from the fastapi module and create an instance of the FastAPI class. This will be your main application object.
- Use the app object to define a path operation, which is a function that handles a request to a specific path. You can use the app.get decorator to specify that the function will handle GET requests to the root path (/).
- Write the logic of the function, which in this case will return a simple JSON response with a message.
- Save the file and run it with Uvicorn, a fast ASGI server that will serve your application. You can use the following command in your terminal:
uvicorn main:app --reload
This will run your application on http://localhost:8000 and reload it automatically whenever you make changes to the code.
The code for the simple API looks like this:
from fastapi import FastAPI app = FastAPI() @app.get("/") def hello(): return {"message": "Hello, world!"}
You can test your API by visiting http://localhost:8000 in your browser or using a tool like curl or Postman. You should see a JSON response like this:
{"message": "Hello, world!"}
Congratulations, you have created your first API with FastAPI!
3. What is Data Validation?
Data validation is the process of checking the data you receive from clients or send back to them for errors, inconsistencies, or violations of certain rules or standards. Data validation helps you ensure that your data is correct, complete, and secure.
In this section, you will learn more about data validation and why it is important for web APIs. You will also learn how FastAPI uses Pydantic to perform data validation and serialization.
Why Data Validation?
Data validation is essential for web APIs for several reasons, such as:
- Quality: Data validation helps you maintain the quality and integrity of your data. It prevents you from accepting invalid or corrupted data that could cause errors or inconsistencies in your application logic or database.
- Security: Data validation helps you protect your data and your application from malicious attacks. It prevents you from accepting data that could contain harmful code or commands that could compromise your system or expose sensitive information.
- User Experience: Data validation helps you provide a better user experience for your clients. It allows you to send back meaningful and informative error messages if the data is invalid, instead of generic or cryptic ones. It also allows you to send back data that is properly formatted and structured for the client’s needs.
These are just some of the benefits of data validation for web APIs. If you want to learn more about data validation and its best practices, you can visit the W3C website or the MDN web docs.
How FastAPI Uses Pydantic for Data Validation?
FastAPI uses Pydantic to perform data validation and serialization for your web API. Pydantic is a library that allows you to define data models with type annotations and perform data validation and serialization. Pydantic has many features that make it a powerful and convenient tool for data validation, such as:
- Type Checking: Pydantic checks the type of the data you receive or send and converts it to the appropriate Python type. For example, if you receive a string that represents a number, Pydantic will convert it to an int or a float, depending on the format.
- Data Conversion: Pydantic also converts the data you receive or send to the appropriate format for the client or the server. For example, if you receive a datetime object, Pydantic will convert it to a string in ISO 8601 format, which is a standard format for date and time.
- Data Validation: Pydantic also validates the data you receive or send against certain rules or constraints that you define in your data model. For example, you can specify the minimum and maximum length of a string, the range of a number, the format of an email, or the presence of a required field.
- Data Serialization: Pydantic also serializes the data you receive or send to a JSON-compatible format, which is the most common format for web APIs. Pydantic handles the serialization and deserialization of complex data types, such as enums, unions, lists, dicts, and nested models.
FastAPI integrates Pydantic seamlessly and gives you access to all its features. You can use Pydantic to define your data models, declare your path operations with your models, and test the validation and serialization of your data.
4. How to Validate Data with FastAPI
In this section, you will learn how to use FastAPI and Pydantic to validate the data you receive from clients or send back to them. You will also learn how to define your data models, declare your path operations with your models, and test the validation and serialization of your data.
How to Define a Pydantic Model?
A Pydantic model is a class that defines the shape and properties of your data. You can use type annotations to specify the type and constraints of each attribute of your model. For example, you can define a model for a user as follows:
from pydantic import BaseModel, EmailStr class User(BaseModel): name: str email: EmailStr age: int is_active: bool
This model has four attributes: name, email, age, and is_active. Each attribute has a type annotation that indicates the type of the data. For example, name is a str, email is an EmailStr, age is an int, and is_active is a bool. EmailStr is a special type provided by Pydantic that validates that the data is a valid email address.
You can also use type annotations to define more complex types, such as enums, unions, lists, dicts, and nested models. For example, you can define a model for a blog post as follows:
from pydantic import BaseModel, HttpUrl from typing import List, Optional from enum import Enum class Category(str, Enum): tech = "tech" lifestyle = "lifestyle" business = "business" class Post(BaseModel): title: str content: str url: HttpUrl category: Category tags: Optional[List[str]] = None author: User
This model has six attributes: title, content, url, category, tags, and author. Each attribute has a type annotation that indicates the type of the data. For example, title is a str, url is a HttpUrl, category is a Category, tags is an optional list of strings, and author is a User. HttpUrl is a special type provided by Pydantic that validates that the data is a valid URL. Category is an enum that defines the possible values for the category attribute. User is a nested model that references the User model defined earlier.
By defining your data models with Pydantic, you can take advantage of its features for data validation and serialization. You can also use your models to declare your path operations with FastAPI.
How to Declare a Path Operation with a Model?
A path operation is a function that handles a request to a specific path. You can use the app object to define a path operation with a decorator that specifies the HTTP method and the path. For example, you can define a path operation that handles GET requests to /users/{user_id} as follows:
@app.get("/users/{user_id}") def get_user(user_id: int): # some logic to get the user by id
This path operation has one parameter: user_id, which is an int that corresponds to the user_id path parameter in the URL. FastAPI will automatically validate that the user_id is an int and convert it to the appropriate Python type.
You can also use your Pydantic models to declare your path operation parameters and responses. For example, you can define a path operation that handles POST requests to /posts as follows:
@app.post("/posts", response_model=Post) def create_post(post: Post): # some logic to create a new post
This path operation has two parameters: post and response_model. The post parameter is a Pydantic model that corresponds to the request body. FastAPI will automatically validate that the request body matches the shape and properties of the Post model and convert it to the appropriate Python object. The response_model parameter is also a Pydantic model that corresponds to the response body. FastAPI will automatically serialize the response body to match the shape and properties of the Post model and convert it to the appropriate JSON format.
By declaring your path operations with your models, you can take advantage of FastAPI’s features for data validation and serialization. You can also use the documentation tools to explore and test your API.
How to Test the Validation and Serialization of Your Data?
FastAPI provides two web-based tools that allow you to interact with your API and test its functionality: Swagger UI and ReDoc. These tools are automatically generated from your code and your models, based on the OpenAPI schema of your API. You can access them by visiting the following URLs:
- Swagger UI: http://localhost:8000/docs
- ReDoc: http://localhost:8000/redoc
These tools allow you to see the details of your endpoints, parameters, responses, and models. You can also use them to send requests to your API and see the responses. For example, you can use Swagger UI to test the validation and serialization of your data as follows:
- Visit http://localhost:8000/docs in your browser.
- Click on the /posts endpoint and then click on the Try it out button.
- Enter some data for the request body, such as:
{ "title": "How to Validate Data with FastAPI", "content": "In this tutorial, you will learn how to use FastAPI and Pydantic to validate the data you receive from clients or send back to them.", "url": "https://example.com/how-to-validate-data-with-fastapi", "category": "tech", "tags": [ "fastapi", "pydantic", "data validation" ], "author": { "name": "Alice", "email": "alice@example.com", "age": 25, "is_active": true } }
- Click on the Execute button and see the response.
You should see a response code of 200 and a response body that matches the data you entered, but with some conversions and validations applied. For example, the url attribute is converted to a string, the category attribute is converted to an enum, and the email attribute is validated to be a valid email address.
You can also try to enter some invalid data and see the error messages. For example, if you enter a non-numeric value for the age attribute, you should see a response code of 422 and a response body that contains the validation error, such as:
{ "detail": [ { "loc": [ "body", "author", "age" ], "msg": "value is not a valid integer", "type": "type_error.integer" } ] }
By using the documentation tools, you can test the validation and serialization of your data and see how your API works.
4.1. Define a Pydantic Model
A Pydantic model is a class that defines the shape and properties of your data. You can use type annotations to specify the type and constraints of each attribute of your model. For example, you can define a model for a user as follows:
from pydantic import BaseModel, EmailStr class User(BaseModel): name: str email: EmailStr age: int is_active: bool
This model has four attributes: name, email, age, and is_active. Each attribute has a type annotation that indicates the type of the data. For example, name is a str, email is an EmailStr, age is an int, and is_active is a bool. EmailStr is a special type provided by Pydantic that validates that the data is a valid email address.
You can also use type annotations to define more complex types, such as enums, unions, lists, dicts, and nested models. For example, you can define a model for a blog post as follows:
from pydantic import BaseModel, HttpUrl from typing import List, Optional from enum import Enum class Category(str, Enum): tech = "tech" lifestyle = "lifestyle" business = "business" class Post(BaseModel): title: str content: str url: HttpUrl category: Category tags: Optional[List[str]] = None author: User
This model has six attributes: title, content, url, category, tags, and author. Each attribute has a type annotation that indicates the type of the data. For example, title is a str, url is a HttpUrl, category is a Category, tags is an optional list of strings, and author is a User. HttpUrl is a special type provided by Pydantic that validates that the data is a valid URL. Category is an enum that defines the possible values for the category attribute. User is a nested model that references the User model defined earlier.
By defining your data models with Pydantic, you can take advantage of its features for data validation and serialization. You can also use your models to declare your path operations with FastAPI.
4.2. Declare a Path Operation with a Model
A path operation is a function that handles a request to a specific path. You can use the app object to define a path operation with a decorator that specifies the HTTP method and the path. For example, you can define a path operation that handles GET requests to /users/{user_id} as follows:
@app.get("/users/{user_id}") def get_user(user_id: int): # some logic to get the user by id
This path operation has one parameter: user_id, which is an int that corresponds to the user_id path parameter in the URL. FastAPI will automatically validate that the user_id is an int and convert it to the appropriate Python type.
You can also use your Pydantic models to declare your path operation parameters and responses. For example, you can define a path operation that handles POST requests to /posts as follows:
@app.post("/posts", response_model=Post) def create_post(post: Post): # some logic to create a new post
This path operation has two parameters: post and response_model. The post parameter is a Pydantic model that corresponds to the request body. FastAPI will automatically validate that the request body matches the shape and properties of the Post model and convert it to the appropriate Python object. The response_model parameter is also a Pydantic model that corresponds to the response body. FastAPI will automatically serialize the response body to match the shape and properties of the Post model and convert it to the appropriate JSON format.
By declaring your path operations with your models, you can take advantage of FastAPI’s features for data validation and serialization. You can also use the documentation tools to explore and test your API.
4.3. Test the Validation
FastAPI provides two web-based tools that allow you to interact with your API and test its functionality: Swagger UI and ReDoc. These tools are automatically generated from your code and your models, based on the OpenAPI schema of your API. You can access them by visiting the following URLs:
- Swagger UI: http://localhost:8000/docs
- ReDoc: http://localhost:8000/redoc
These tools allow you to see the details of your endpoints, parameters, responses, and models. You can also use them to send requests to your API and see the responses. For example, you can use Swagger UI to test the validation and serialization of your data as follows:
- Visit http://localhost:8000/docs in your browser.
- Click on the /posts endpoint and then click on the Try it out button.
- Enter some data for the request body, such as:
{ "title": "How to Validate Data with FastAPI", "content": "In this tutorial, you will learn how to use FastAPI and Pydantic to validate the data you receive from clients or send back to them.", "url": "https://example.com/how-to-validate-data-with-fastapi", "category": "tech", "tags": [ "fastapi", "pydantic", "data validation" ], "author": { "name": "Alice", "email": "alice@example.com", "age": 25, "is_active": true } }
- Click on the Execute button and see the response.
You should see a response code of 200 and a response body that matches the data you entered, but with some conversions and validations applied. For example, the url attribute is converted to a string, the category attribute is converted to an enum, and the email attribute is validated to be a valid email address.
You can also try to enter some invalid data and see the error messages. For example, if you enter a non-numeric value for the age attribute, you should see a response code of 422 and a response body that contains the validation error, such as:
{ "detail": [ { "loc": [ "body", "author", "age" ], "msg": "value is not a valid integer", "type": "type_error.integer" } ] }
By using the documentation tools, you can test the validation and serialization of your data and see how your API works.
5. What is OpenAPI Schema?
OpenAPI schema is a standard and language-agnostic way of describing the structure and behavior of your web API. It is based on the OpenAPI Specification, which is a widely adopted and supported standard for designing and documenting web APIs. OpenAPI schema allows you to define the endpoints, parameters, responses, and models of your API in a JSON or YAML format. For example, you can define a simple OpenAPI schema for your API as follows:
openapi: 3.0.0 info: title: FastAPI Validation and Documentation version: 1.0.0 paths: /users/{user_id}: get: summary: Get a user by id parameters: - name: user_id in: path required: true schema: type: integer responses: 200: description: A user object content: application/json: schema: $ref: '#/components/schemas/User' 404: description: User not found /posts: post: summary: Create a new post requestBody: required: true content: application/json: schema: $ref: '#/components/schemas/Post' responses: 200: description: A post object content: application/json: schema: $ref: '#/components/schemas/Post' components: schemas: User: type: object properties: name: type: string email: type: string format: email age: type: integer is_active: type: boolean Post: type: object properties: title: type: string content: type: string url: type: string format: uri category: type: string enum: [tech, lifestyle, business] tags: type: array items: type: string author: $ref: '#/components/schemas/User'
By defining your OpenAPI schema, you can take advantage of several benefits, such as:
- Documentation: OpenAPI schema allows you to generate interactive documentation for your API automatically, using tools like Swagger UI and ReDoc. You can use the documentation tools to explore and test your API without writing any extra code.
- Validation: OpenAPI schema allows you to validate the data you receive or send against the schema and send back meaningful error messages if the data is invalid. You can use tools like FastAPI to perform the validation and serialization automatically, based on your schema.
- Interoperability: OpenAPI schema allows you to communicate the structure and behavior of your API to other developers or applications in a standard and language-agnostic way. You can use tools like OpenAPI Generator to generate client or server code for your API in different languages, based on your schema.
These are just some of the benefits of OpenAPI schema for web APIs. If you want to learn more about OpenAPI schema and its features, you can visit the official website or the GitHub repository.
6. How to Generate OpenAPI Schema with FastAPI
One of the great features of FastAPI is that it automatically generates an OpenAPI schema for your API, based on your code and your models. You don’t have to write any extra code or configuration to create your OpenAPI schema. FastAPI does it for you behind the scenes.
In this section, you will learn how to access and customize your OpenAPI schema with FastAPI. You will also learn how to use the documentation tools to explore and test your API based on your OpenAPI schema.
How to Access Your OpenAPI Schema?
To access your OpenAPI schema, you can visit the following URL in your browser:
- http://localhost:8000/openapi.json
This will show you the JSON representation of your OpenAPI schema, which describes the endpoints, parameters, responses, and models of your API. You can also access the YAML representation of your OpenAPI schema by visiting the following URL:
- http://localhost:8000/openapi.yaml
You can use these URLs to download or share your OpenAPI schema with other developers or applications. You can also use them to generate client or server code for your API in different languages, using tools like OpenAPI Generator.
How to Customize Your OpenAPI Schema?
FastAPI provides some options to customize your OpenAPI schema, such as:
- Title: You can set the title of your API by passing the title parameter to the FastAPI class. For example:
app = FastAPI(title="FastAPI Validation and Documentation")
- Version: You can set the version of your API by passing the version parameter to the FastAPI class. For example:
app = FastAPI(version="1.0.0")
- Description: You can set the description of your API by passing the description parameter to the FastAPI class. You can use Markdown syntax to format your description. For example:
app = FastAPI(description="This is a tutorial on how to use FastAPI and Pydantic to validate the data you receive from clients or send back to them.")
- Summary: You can set the summary of each path operation by passing the summary parameter to the path operation decorator. This will show a brief description of the path operation in the documentation tools. For example:
@app.get("/users/{user_id}", summary="Get a user by id") def get_user(user_id: int): # some logic to get the user by id
- Tags: You can set the tags of each path operation by passing the tags parameter to the path operation decorator. This will group the path operations by tags in the documentation tools. For example:
@app.post("/posts", response_model=Post, tags=["posts"], summary="Create a new post") def create_post(post: Post): # some logic to create a new post
These are just some of the options to customize your OpenAPI schema with FastAPI. If you want to learn more about the options and how to use them, you can visit the official documentation.
7. What is Swagger UI?
Swagger UI is a web-based tool that allows you to interact with your web API and test its functionality. It is based on the OpenAPI schema of your API, which describes the endpoints, parameters, responses, and models of your API. Swagger UI generates interactive documentation for your API automatically, using the information from your OpenAPI schema.
In this section, you will learn how to use Swagger UI with FastAPI. You will also learn how to customize the appearance and behavior of Swagger UI.
How to Use Swagger UI with FastAPI?
To use Swagger UI with FastAPI, you don’t have to do anything special. FastAPI integrates with Swagger UI by default and provides a URL for you to access it. You can visit the following URL in your browser:
- http://localhost:8000/docs
This will show you the Swagger UI interface, which looks something like this:
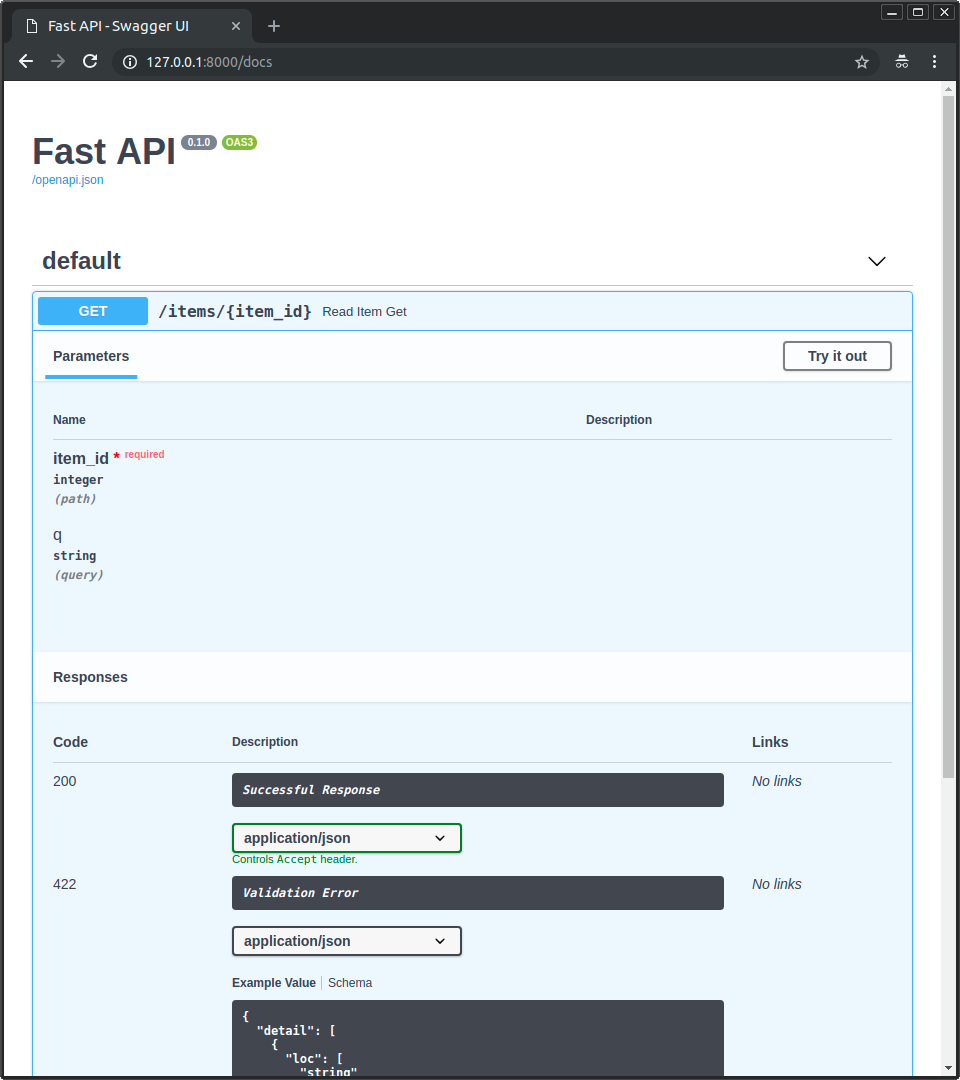
You can see the details of your endpoints, parameters, responses, and models in the Swagger UI interface. You can also use the interface to send requests to your API and see the responses. For example, you can use Swagger UI to test the validation and serialization of your data as follows:
- Click on the /posts endpoint and then click on the Try it out button.
- Enter some data for the request body, such as:
{ "title": "How to Validate Data with FastAPI", "content": "In this tutorial, you will learn how to use FastAPI and Pydantic to validate the data you receive from clients or send back to them.", "url": "https://example.com/how-to-validate-data-with-fastapi", "category": "tech", "tags": [ "fastapi", "pydantic", "data validation" ], "author": { "name": "Alice", "email": "alice@example.com", "age": 25, "is_active": true } }
- Click on the Execute button and see the response.
You should see a response code of 200 and a response body that matches the data you entered, but with some conversions and validations applied. For example, the url attribute is converted to a string, the category attribute is converted to an enum, and the email attribute is validated to be a valid email address.
You can also try to enter some invalid data and see the error messages. For example, if you enter a non-numeric value for the age attribute, you should see a response code of 422 and a response body that contains the validation error, such as:
{ "detail": [ { "loc": [ "body", "author", "age" ], "msg": "value is not a valid integer", "type": "type_error.integer" } ] }
By using Swagger UI, you can test the validation and serialization of your data and see how your API works.
8. How to Use Swagger UI with FastAPI
Swagger UI is a web-based tool that allows you to interact with your web API and test its functionality. It is based on the OpenAPI schema of your API, which describes the endpoints, parameters, responses, and models of your API. Swagger UI generates interactive documentation for your API automatically, using the information from your OpenAPI schema.
In this section, you will learn how to use Swagger UI with FastAPI. You will also learn how to customize the appearance and behavior of Swagger UI.
How to Use Swagger UI with FastAPI?
To use Swagger UI with FastAPI, you don’t have to do anything special. FastAPI integrates with Swagger UI by default and provides a URL for you to access it. You can visit the following URL in your browser:
- http://localhost:8000/docs
This will show you the Swagger UI interface, which looks something like this:
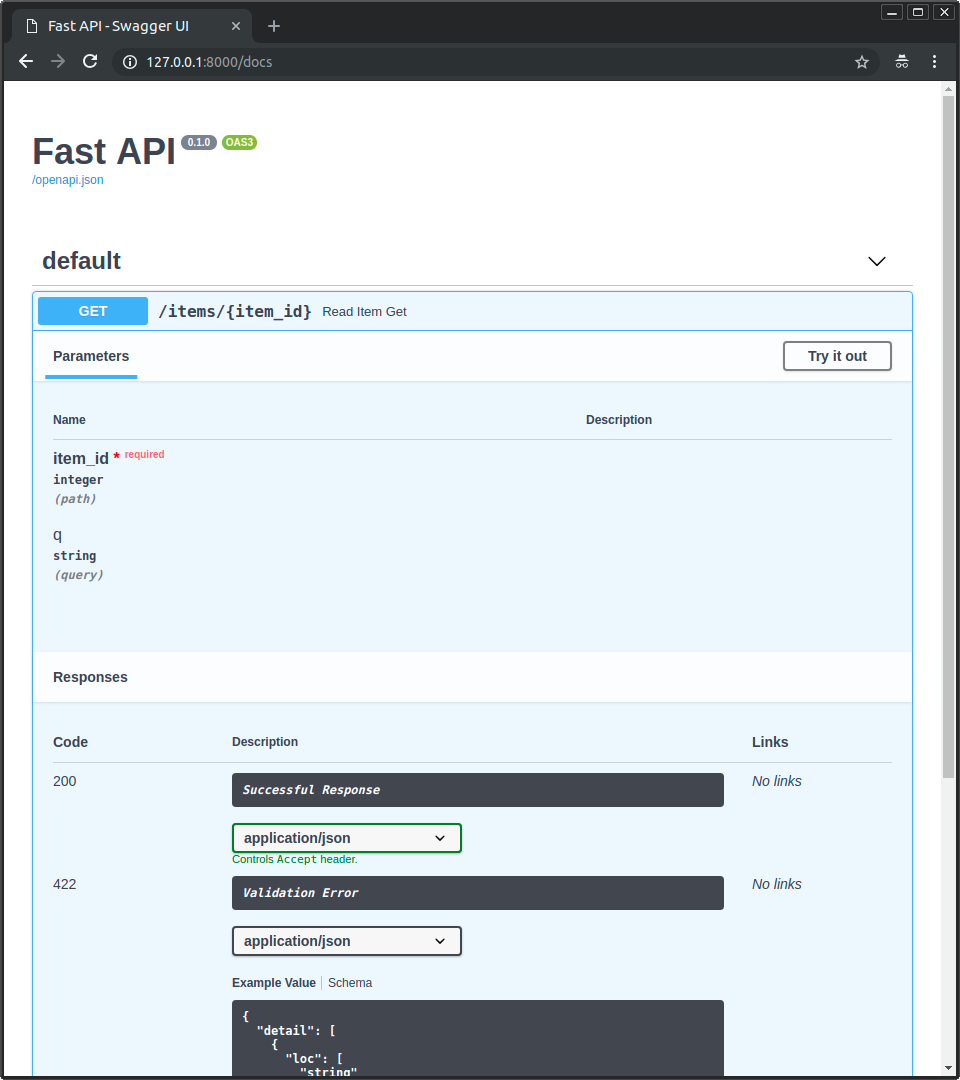
You can see the details of your endpoints, parameters, responses, and models in the Swagger UI interface. You can also use the interface to send requests to your API and see the responses. For example, you can use Swagger UI to test the validation and serialization of your data as follows:
- Click on the /posts endpoint and then click on the Try it out button.
- Enter some data for the request body, such as:
{ "title": "How to Validate Data with FastAPI", "content": "In this tutorial, you will learn how to use FastAPI and Pydantic to validate the data you receive from clients or send back to them.", "url": "https://example.com/how-to-validate-data-with-fastapi", "category": "tech", "tags": [ "fastapi", "pydantic", "data validation" ], "author": { "name": "Alice", "email": "alice@example.com", "age": 25, "is_active": true } }
- Click on the Execute button and see the response.
You should see a response code of 200 and a response body that matches the data you entered, but with some conversions and validations applied. For example, the url attribute is converted to a string, the category attribute is converted to an enum, and the email attribute is validated to be a valid email address.
You can also try to enter some invalid data and see the error messages. For example, if you enter a non-numeric value for the age attribute, you should see a response code of 422 and a response body that contains the validation error, such as:
{ "detail": [ { "loc": [ "body", "author", "age" ], "msg": "value is not a valid integer", "type": "type_error.integer" } ] }
By using Swagger UI, you can test the validation and serialization of your data and see how your API works.
How to Customize Swagger UI?
FastAPI also provides some options to customize Swagger UI, such as:
- Docs URL: You can change the URL where Swagger UI is served by passing the docs_url parameter to the FastAPI class. For example, if you want to serve Swagger UI at http://localhost:8000/api/docs, you can do:
app = FastAPI(docs_url="/api/docs")
- Swagger UI Config: You can pass a dictionary of configuration options to the swagger_ui_init_oauth parameter of the FastAPI class. This will allow you to customize the appearance and behavior of Swagger UI. For example, if you want to change the theme of Swagger UI, you can do:
app = FastAPI(swagger_ui_init_oauth={"theme": "monokai"})
You can find the list of available configuration options in the official documentation.
- Custom CSS: You can also add custom CSS styles to Swagger UI by passing the swagger_ui_css parameter to the FastAPI class. This will allow you to override the default styles of Swagger UI. For example, if you want to change the font size of Swagger UI, you can do:
app = FastAPI(swagger_ui_css=".swagger-ui * {font-size: 16px;}")
These are just some of the options to customize Swagger UI with FastAPI. If you want to learn more about the options and how to use them, you can visit the official documentation.
9. What is ReDoc?
ReDoc is another web-based tool that allows you to generate interactive documentation for your web API, based on your OpenAPI schema. ReDoc is similar to Swagger UI, but it has a different design and layout. ReDoc provides a more elegant and customizable documentation for your API, with features such as:
- Side menu: ReDoc displays a side menu that shows the tags and endpoints of your API, along with the descriptions and examples. You can use the side menu to navigate through your API and see the details of each endpoint.
- Three-panel view: ReDoc shows a three-panel view that shows the request, response, and schema of each endpoint. You can use the three-panel view to see the parameters, headers, body, and examples of each request and response. You can also see the schema and model of each data type.
- Code samples: ReDoc generates code samples for each endpoint in different languages, such as Python, JavaScript, Ruby, and more. You can use the code samples to see how to call your API from different clients and platforms.
- Search: ReDoc provides a search feature that allows you to search for keywords or phrases in your API documentation. You can use the search feature to find the information you need quickly and easily.
In this section, you will learn how to use ReDoc with FastAPI. You will also learn how to customize the appearance and behavior of ReDoc.
10. How to Use ReDoc with FastAPI
ReDoc is another web-based tool that allows you to generate interactive documentation for your web API, based on your OpenAPI schema. ReDoc is similar to Swagger UI, but it has a different design and layout. ReDoc provides a more elegant and customizable documentation for your API, with features such as:
- Side menu: ReDoc displays a side menu that shows the tags and endpoints of your API, along with the descriptions and examples. You can use the side menu to navigate through your API and see the details of each endpoint.
- Three-panel view: ReDoc shows a three-panel view that shows the request, response, and schema of each endpoint. You can use the three-panel view to see the parameters, headers, body, and examples of each request and response. You can also see the schema and model of each data type.
- Code samples: ReDoc generates code samples for each endpoint in different languages, such as Python, JavaScript, Ruby, and more. You can use the code samples to see how to call your API from different clients and platforms.
- Search: ReDoc provides a search feature that allows you to search for keywords or phrases in your API documentation. You can use the search feature to find the information you need quickly and easily.
In this section, you will learn how to use ReDoc with FastAPI. You will also learn how to customize the appearance and behavior of ReDoc.
How to Use ReDoc with FastAPI?
To use ReDoc with FastAPI, you don’t have to do anything special. FastAPI integrates with ReDoc by default and provides a URL for you to access it. You can visit the following URL in your browser:
- http://localhost:8000/redoc
This will show you the ReDoc interface, which looks something like this:
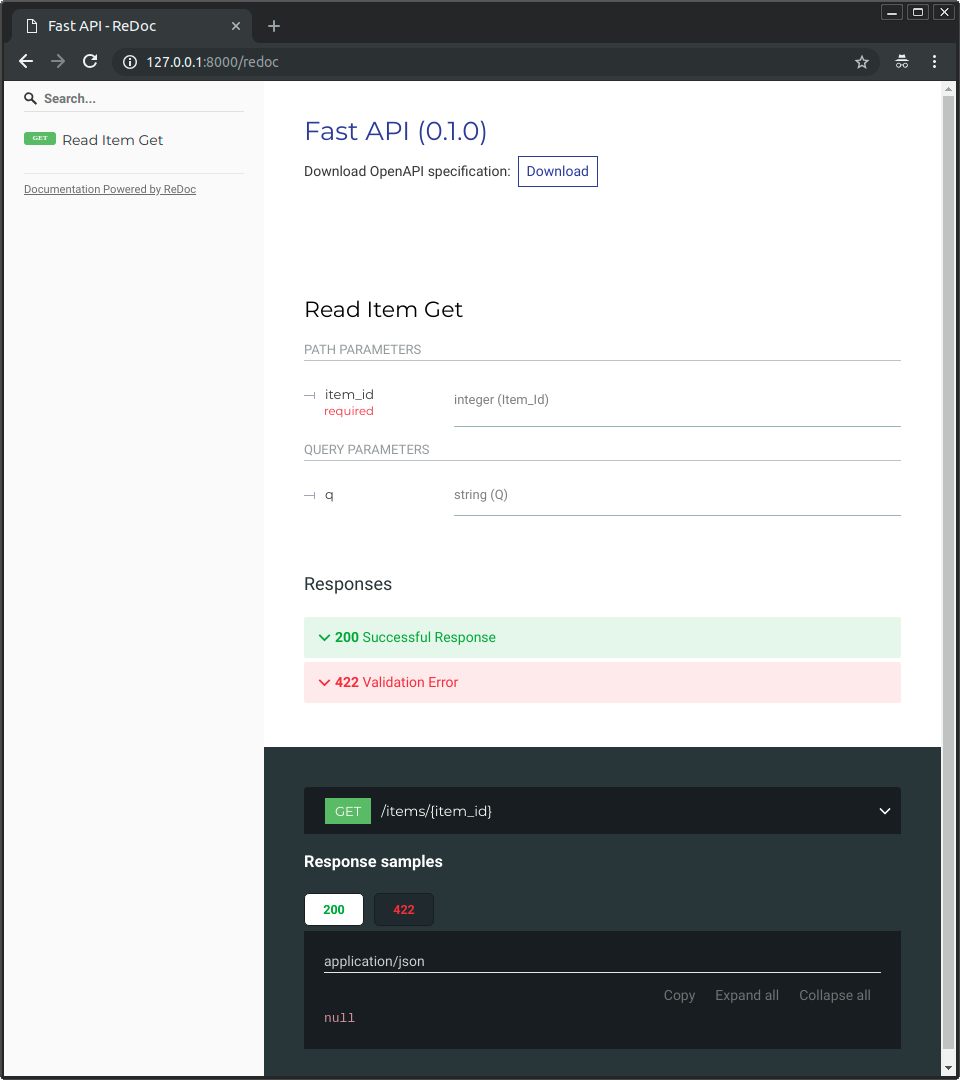
You can see the details of your endpoints, parameters, responses, and models in the ReDoc interface. You can also use the interface to see the examples and code samples of each endpoint. However, unlike Swagger UI, ReDoc does not allow you to send requests to your API and see the responses. ReDoc is more focused on providing a static and elegant documentation for your API, rather than an interactive one.
How to Customize ReDoc?
FastAPI also provides some options to customize ReDoc, such as:
- ReDoc URL: You can change the URL where ReDoc is served by passing the redoc_url parameter to the FastAPI class. For example, if you want to serve ReDoc at http://localhost:8000/api/redoc, you can do:
app = FastAPI(redoc_url="/api/redoc")
- ReDoc Config: You can pass a dictionary of configuration options to the redoc_configs parameter of the FastAPI class. This will allow you to customize the appearance and behavior of ReDoc. For example, if you want to hide the loading animation of ReDoc, you can do:
app = FastAPI(redoc_configs={"hideLoading": True})
You can find the list of available configuration options in the official documentation.
- Custom CSS: You can also add custom CSS styles to ReDoc by passing the redoc_css parameter to the FastAPI class. This will allow you to override the default styles of ReDoc. For example, if you want to change the background color of ReDoc, you can do:
app = FastAPI(redoc_css="body {background-color: lightblue;}")
These are just some of the options to customize ReDoc with FastAPI. If you want to learn more about the options and how to use them, you can visit the official documentation.
11. Conclusion
In this tutorial, you have learned how to use FastAPI to validate your data and generate interactive documentation for your web API using OpenAPI schema, Swagger UI, and ReDoc. You have also learned how to customize the appearance and behavior of the documentation tools.
FastAPI is a modern, high-performance, web framework for building APIs with Python. It is based on standard Python type hints and the powerful Pydantic library. FastAPI provides several features that make it easy to create and document your web API, such as:
- Data validation: FastAPI validates the data you receive from clients and sends back meaningful error messages if the data is invalid.
- OpenAPI schema: FastAPI automatically generates an OpenAPI schema for your API, which describes the endpoints, parameters, responses, and models of your API.
- Swagger UI: FastAPI integrates with Swagger UI, a web-based tool that allows you to interact with your API and test its functionality.
- ReDoc: FastAPI also integrates with ReDoc, another web-based tool that provides a more elegant and customizable documentation for your API.
By using FastAPI and its features, you can create a web API that is fast, simple, and well-documented. You can also test the validation and serialization of your data and see how your API works.
If you want to learn more about FastAPI and its features, you can visit the official website or the GitHub repository. You can also find more tutorials and examples on the official documentation.
Thank you for reading this tutorial and I hope you found it useful and enjoyable. If you have any questions or feedback, feel free to leave a comment below. Happy coding!